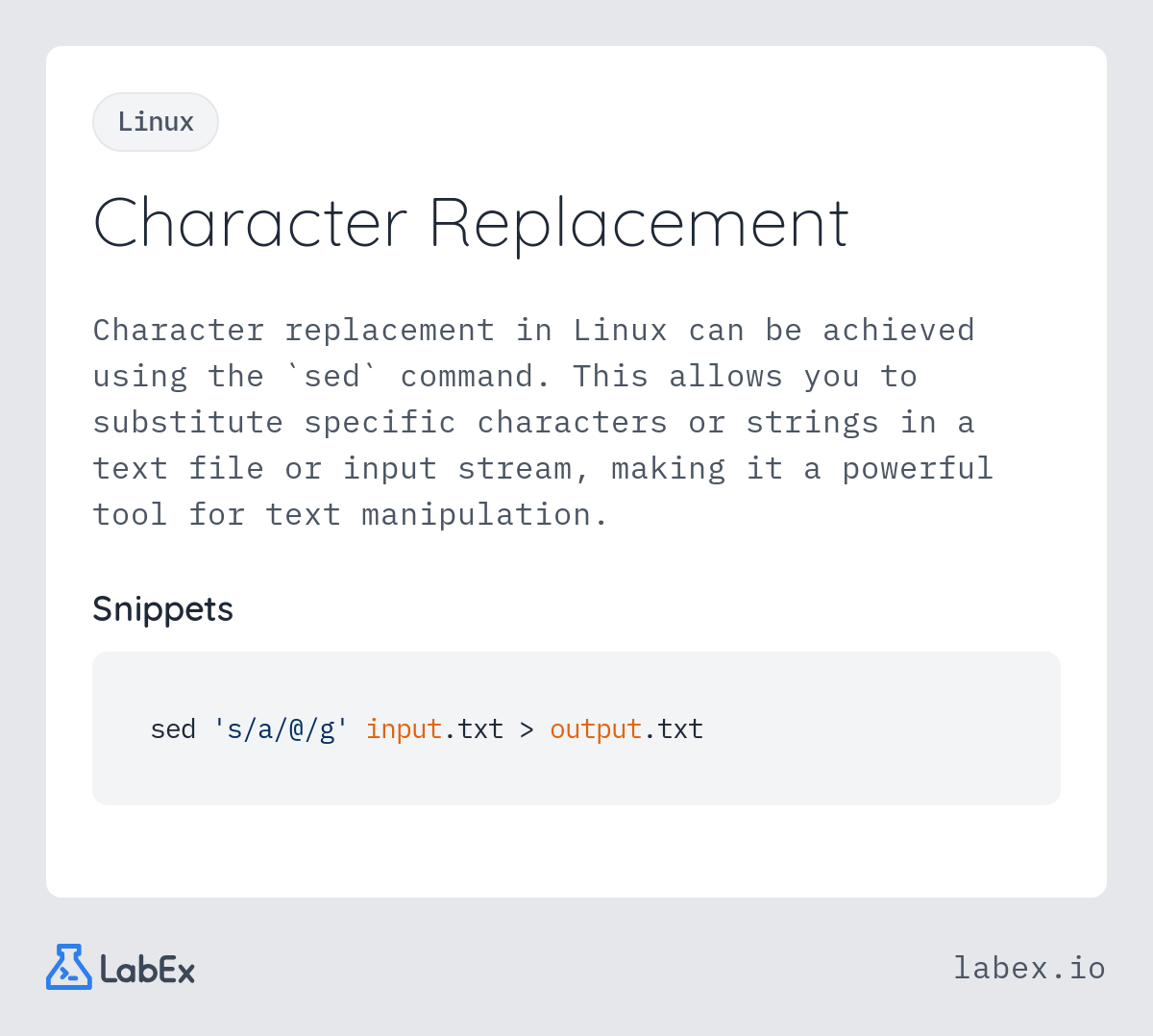
Character Replacement
Character replacement in Linux can be achieved using the `sed` command. This allows you to substitute specific characters or strings in a text file or input stream, making it a powerful tool for text manipulation.
Explore our curated collection of programming flashcards. Each card contains practical examples and code snippets to help you master programming concepts quickly.