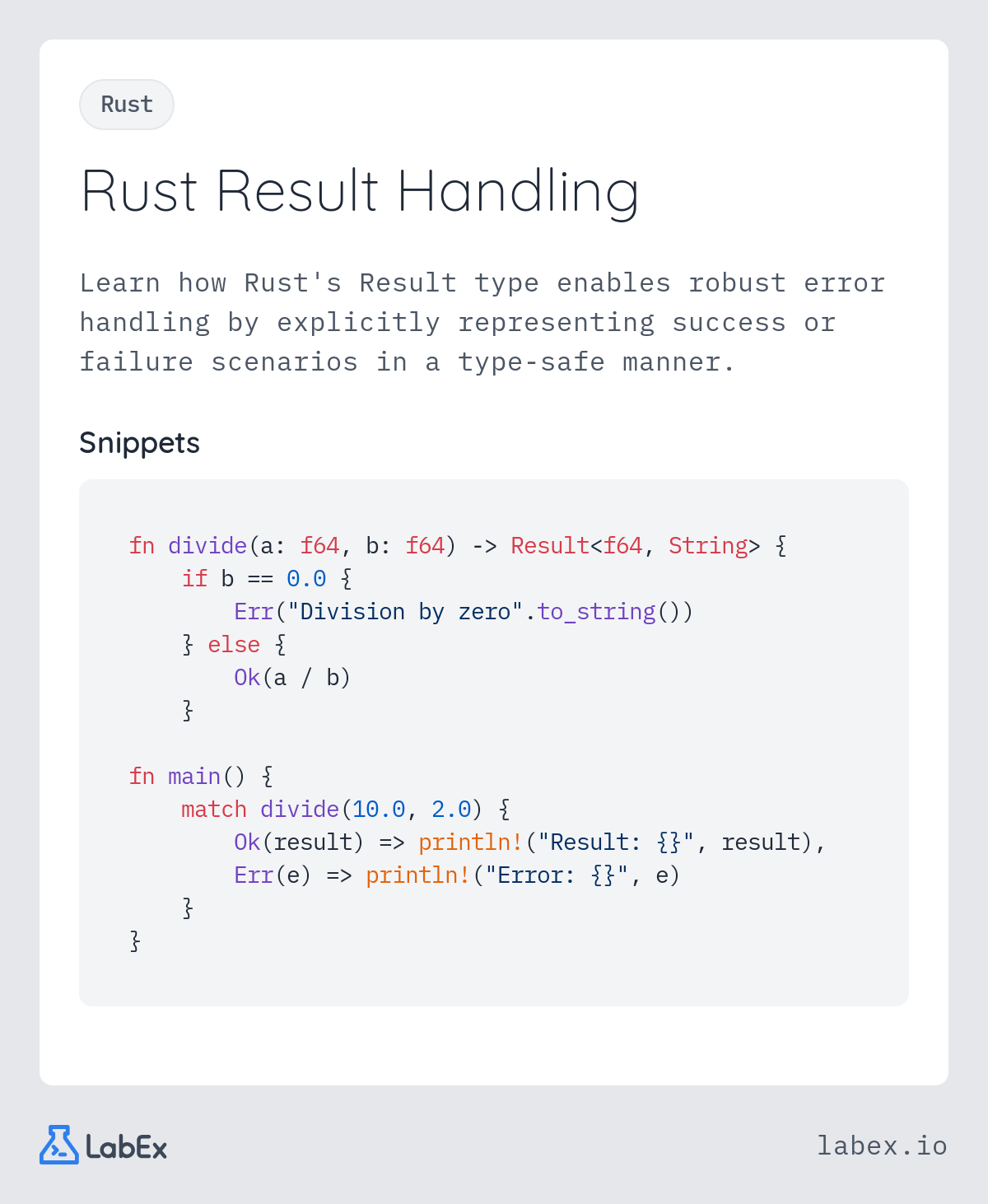
Rust Result Handling
Learn how Rust's Result type enables robust error handling by explicitly representing success or failure scenarios in a type-safe manner.
Explore our curated collection of programming flashcards. Each card contains practical examples and code snippets to help you master programming concepts quickly.