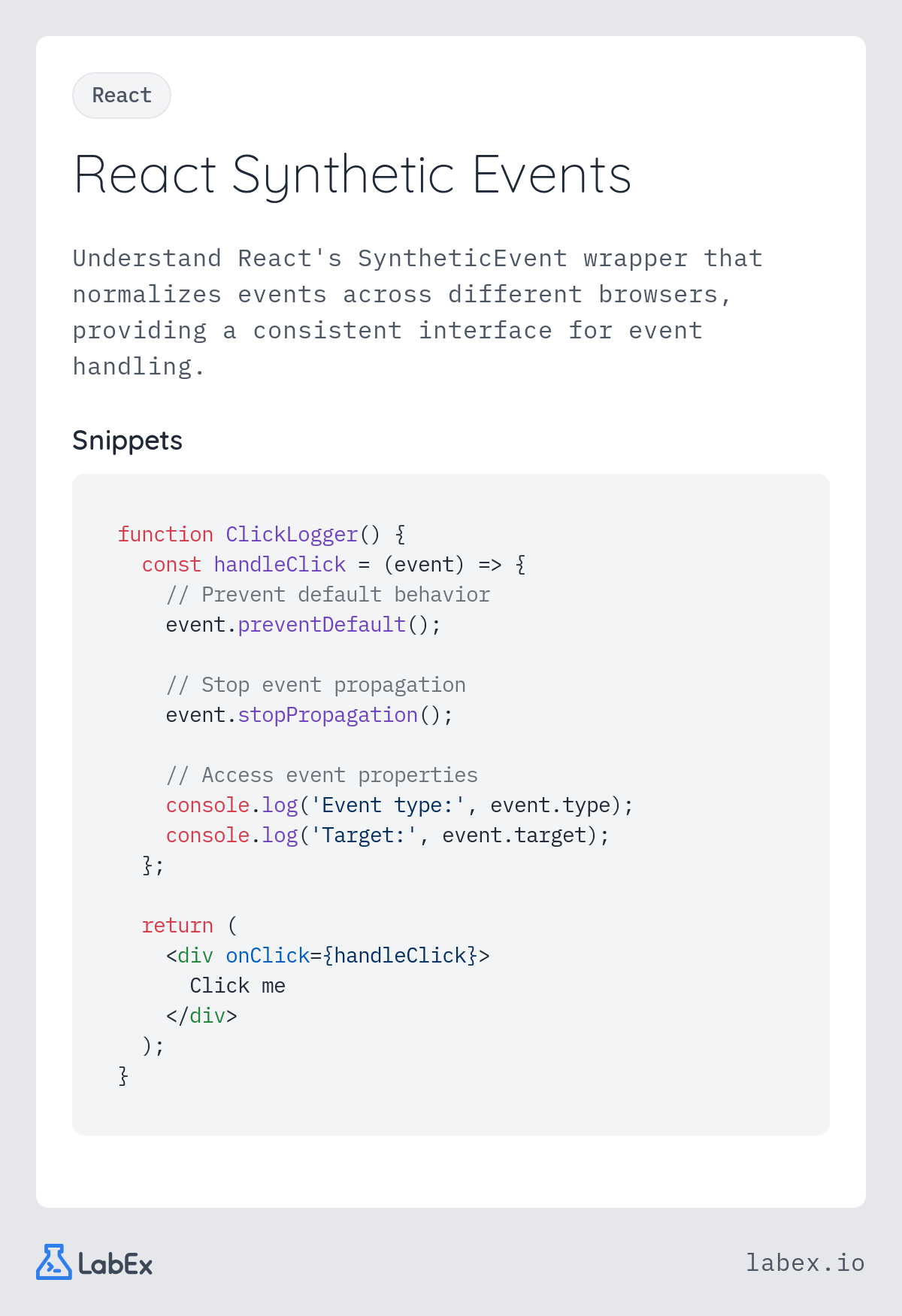
React Synthetic Events
Understand React's SyntheticEvent wrapper that normalizes events across different browsers, providing a consistent interface for event handling.
Explore our curated collection of programming flashcards. Each card contains practical examples and code snippets to help you master programming concepts quickly.