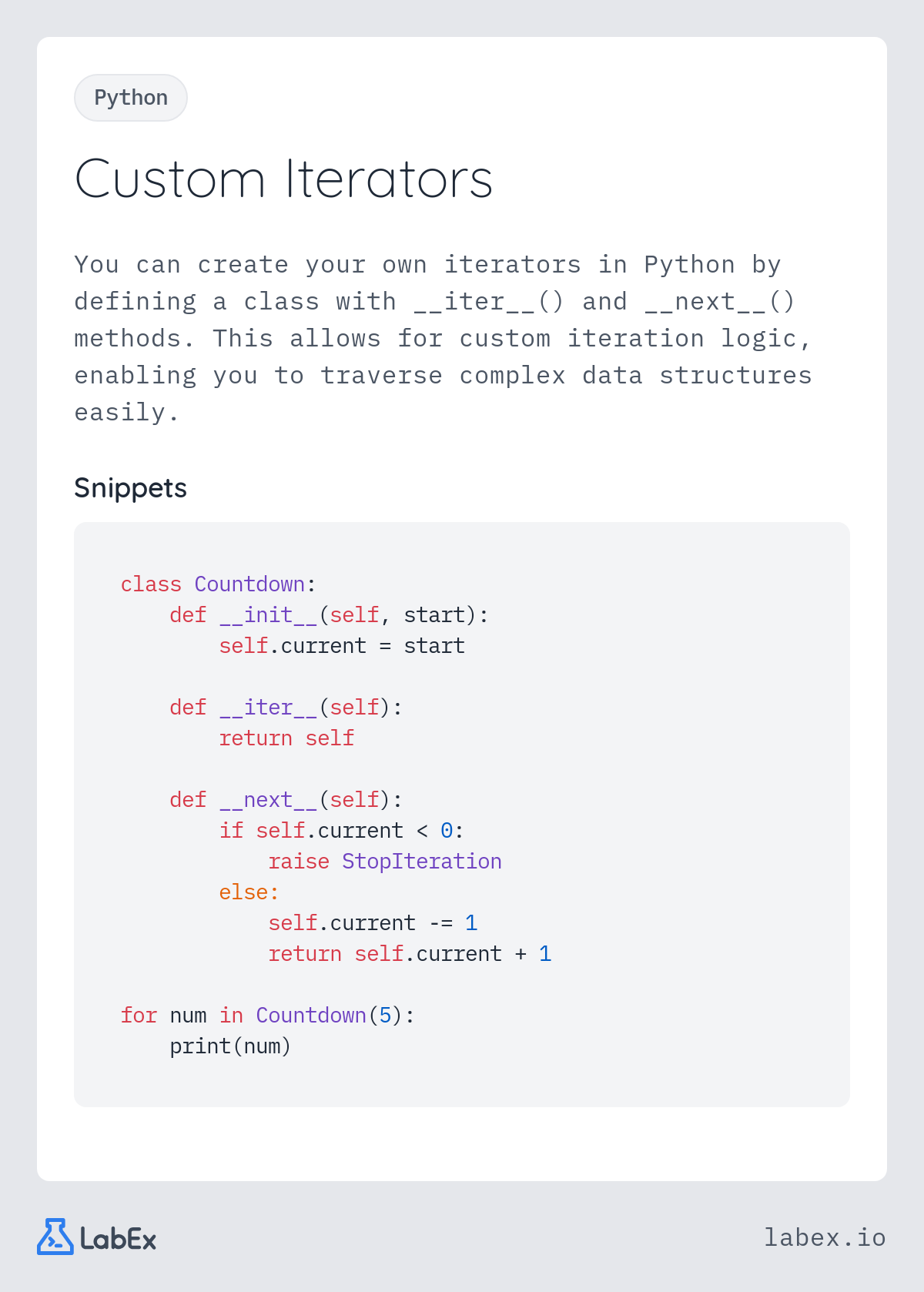
Custom Iterators
You can create your own iterators in Python by defining a class with __iter__() and __next__() methods. This allows for custom iteration logic, enabling you to traverse complex data structures easily.
Explore our curated collection of programming flashcards. Each card contains practical examples and code snippets to help you master programming concepts quickly.