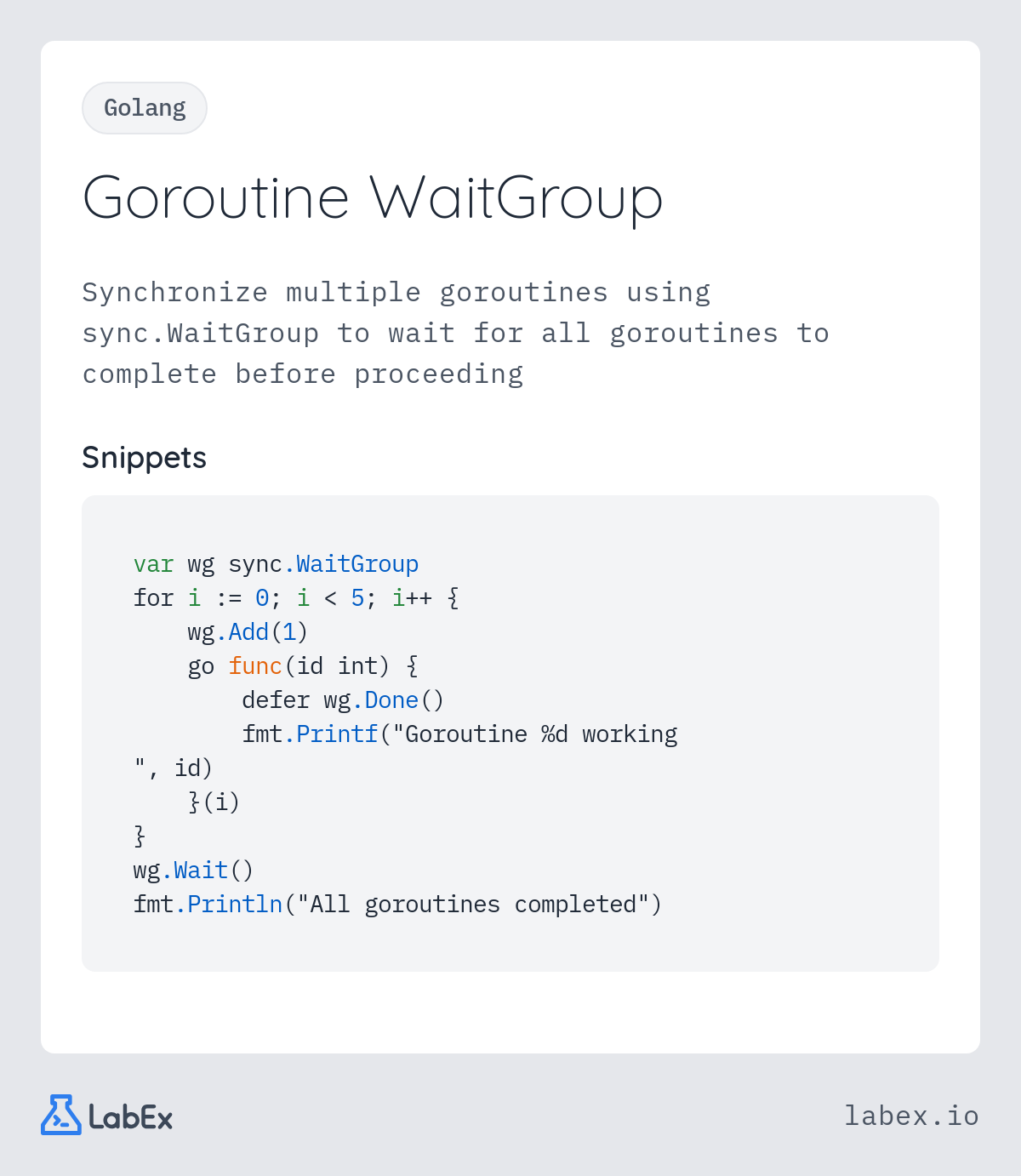
Goroutine WaitGroup
Synchronize multiple goroutines using sync.WaitGroup to wait for all goroutines to complete before proceeding
Explore our curated collection of programming flashcards. Each card contains practical examples and code snippets to help you master programming concepts quickly.